1. Design the form
'Retrofit' the form data string below:
name=Evan+Burke&card=Visa&number=8443261344895544&order=French+perfume
for buying some French perfume into the HTML form fields and submit button on the Web page form.
Source Code for the order form:
<html>
<head>
<title>Order</title>
</head>
<body>
<form action="order.htm" method="get">
<table>
<tr><td>Name: </td><td><input name="name" type="text"/></td></tr>
<tr><td>Card: </td><td><input name="card" type="text" /></td></tr>
<tr><td>Number: </td><td><input name="number" type="text" /></td></tr>
<tr><td>Order: </td><td><input name="order" type="text" /></td></tr>
<tr><td></td><td><input type="submit" value="submit" /></td></tr>
</form>
</body>
</html>
Screenshot:
2. Script archives exist for PERL, Python and JavaScript. Search the Web for a script that processes the HTML forms data. Read the code and list the steps involved in processing the form.
JavaScript:
html>
<head>
<title>Javascript Test</title>
<script language="JavaScript">
function readText (form){
TestVar = form.inputbox.value;
document.write ("You have entered: " +TestVar);
}
</script>
</head>
<body>
<form name = "javascript test" action="" method="get">
Enter test string: <br>
<input type="text" name="inputbox" value=""><P>
<input type="button" name="button1" value="Read" onClick="readText(this.form)">
</form>
</body>
</html>
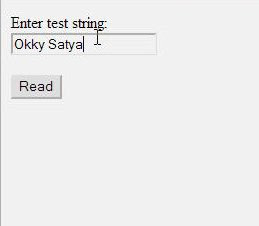
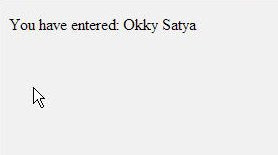
3. Can you modify the script to process the form?
Yes, I could modify the script to process the order form, but it would be futile as in real practice, processing the data on the form would be done by passing the data from the input boxes on to the server and commonly followed on to the database for updating.
For testing purposes, I am modifying the above script to forward the input entered to the form into text labels in the browser
Source Code:
<html>
<head>
<title>Javascript Test</title>
<script language="JavaScript">
function readText(form){
Name=form.nama.value;
Card=form.kartu.value;
Number=form.nomer.value;
Order=form.pilih.value;
document.write ("<p>Name : " + Name + "<br>Card : " + Card + "<br>Number : " + Number + "<br>Order : " + Order +"<p>");
}
</script>
</head>
<body>
<form name = "shop-test" action="" method="get">
<table>
<tr><td>Name: </td><td><input name="nama" type="text"/></td></tr>
<tr><td>Card: </td><td><input name="kartu" type="text" /></td></tr>
<tr><td>Number: </td><td><input name="nomer" type="text" /></td></tr>
<tr><td>Order: </td><td><input name="pilih" type="text" /></td></tr>
<tr><td></td><td><input type="Submit" name="submit1" value="Submit" onClick="readText(this.form)"></td></tr>
</form>
</body>
</html>
Screenshot:
Output:
4. Improve the user experience by add a Javascript feature.
I am adding a drop-down list feature for card selection as well as a number auto-validation to ensure that the entered data in the numbers field are always numeric. This is done by adding the display() function for the drop-down list and function isNumber() to validate the numerical data. In the display() function, users will be prompted by a message box with a reminder to enter their details.
Source Code:
<html>
<head>
<title>Javascript Test</title>
<script language="JavaScript">
function readText(form){
Name=form.nama.value;
Card=form.kartu.value;
Number=form.nomer.value;
Order=form.pilih.value;
document.write ("<p>Name : " + Name + "<br>Card : " + Card + "<br>Number : " + Number + "<br>Order : " + Order +"<p>");
}
function display()
{
var tmp = document.shoptest.kartu.selectedIndex;
var selected_text = document.shoptest.kartu.options[tmp].text;
alert("Your have selected : " +"\n" + selected_text +" Card"+"\n please enter the required details");
}
function IsNumber() {
if (event.keyCode>='0'.charCodeAt()&&event.keyCode <= '9'.charCodeAt())
event.returnValue = true;
else
event.returnValue = false;
}
</script>
</head>
<body>
<form name = "shoptest" action="" method="get">
<table>
<tr><td>Name: </td><td><input name="nama" type="text"/></td></tr>
<tr><td>Card: </td><td><select name="kartu" onChange="display()">
<option value = "VISA">VISA
<option value = "MASTERCARD">MASTERCARD
<option value = "AMEX">AMEX
<option value = "DINERS">DINERS
</select></td></tr>
<tr><td>Number: </td><td><input name="nomer" type="text" onkeypress="return IsNumber(this.name);" /></td></tr>
<tr><td>Order: </td><td><input name="pilih" type="text" /></td></tr>
<tr><td></td><td><input type="Submit" name="submit1" value="Submit" onClick="readText(this.form)"></td></tr>
</form>
</body>
</html>
Screenshot
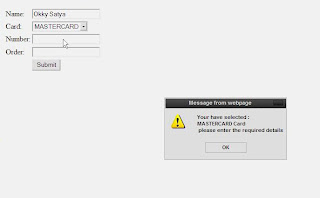
0 comments:
Post a Comment